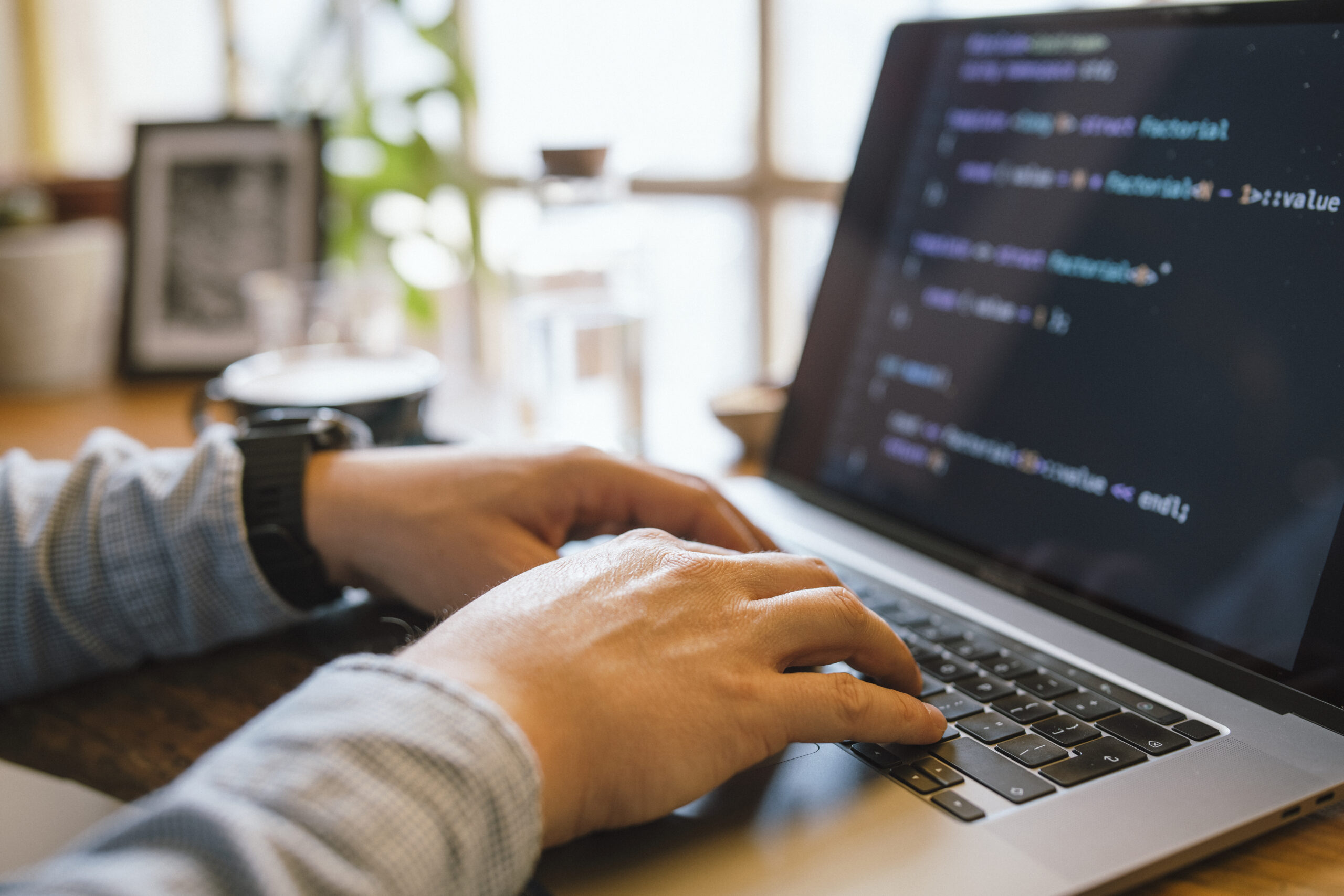
Debugging is Just about the most necessary — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much fixing broken code; it’s about knowing how and why factors go Mistaken, and Mastering to Assume methodically to solve issues efficiently. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging techniques can help save hrs of irritation and radically help your efficiency. Here are several strategies that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest means builders can elevate their debugging expertise is by mastering the instruments they use every single day. Although writing code is a person Component of growth, realizing the way to interact with it correctly all through execution is Similarly essential. Modern development environments occur Outfitted with powerful debugging abilities — but several builders only scratch the area of what these instruments can do.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and also modify code over the fly. When utilised properly, they Permit you to observe particularly how your code behaves for the duration of execution, that is a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for front-close builders. They allow you to inspect the DOM, keep an eye on community requests, check out authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can transform aggravating UI difficulties into workable duties.
For backend or procedure-degree developers, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working processes and memory management. Finding out these instruments may have a steeper Understanding curve but pays off when debugging effectiveness concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfortable with Edition Management units like Git to understand code heritage, come across the precise instant bugs were introduced, and isolate problematic modifications.
Eventually, mastering your applications means going past default options and shortcuts — it’s about building an intimate understanding of your enhancement environment so that when problems arise, you’re not lost at midnight. The higher you recognize your equipment, the more time you'll be able to devote fixing the actual dilemma as an alternative to fumbling by way of the method.
Reproduce the issue
Probably the most critical — and often ignored — actions in effective debugging is reproducing the issue. Before leaping in the code or generating guesses, builders want to create a dependable ecosystem or circumstance in which the bug reliably appears. With out reproducibility, correcting a bug will become a recreation of opportunity, normally resulting in wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Ask issues like: What steps resulted in The difficulty? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact conditions beneath which the bug occurs.
As soon as you’ve collected enough data, try to recreate the situation in your local setting. This could necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The problem seems intermittently, look at writing automated checks that replicate the edge situations or point out transitions concerned. These assessments not just enable expose the problem but in addition reduce regressions in the future.
Often, The difficulty could be natural environment-specific — it might come about only on sure operating techniques, browsers, or underneath specific configurations. Making use of applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the challenge isn’t merely a step — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But after you can persistently recreate the bug, you happen to be now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging equipment far more properly, take a look at probable fixes safely and securely, and converse additional Plainly with your team or users. It turns an summary criticism right into a concrete problem — and that’s exactly where builders thrive.
Go through and Realize the Error Messages
Error messages are often the most valuable clues a developer has when some thing goes Incorrect. Instead of looking at them as disheartening interruptions, builders must find out to treat error messages as immediate communications with the technique. They usually tell you what precisely took place, the place it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly and in total. Numerous builders, particularly when below time tension, glance at the first line and straight away get started generating assumptions. But deeper from the error stack or logs may perhaps lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google — browse and realize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or perhaps a logic mistake? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can tutorial your investigation and stage you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Understanding to acknowledge these can drastically quicken your debugging course of action.
Some errors are imprecise or generic, As well as in These scenarios, it’s very important to examine the context during which the mistake happened. Verify similar log entries, input values, and recent adjustments from the codebase.
Don’t overlook compiler or linter warnings either. These usually precede more substantial challenges and provide hints about probable bugs.
Ultimately, error messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues more rapidly, cut down debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment inside of a developer’s debugging toolkit. When used effectively, it provides real-time insights into how an application behaves, helping you understand what’s happening underneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what level. Popular logging ranges consist of DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, INFO for general situations (like prosperous start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for real problems, and Lethal once the method can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure important messages and decelerate your program. Concentrate on vital functions, state variations, input/output values, and critical decision details within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s easier to trace difficulties in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without having halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a properly-thought-out logging strategy, you may reduce the time it's going to take to spot concerns, get deeper visibility into your programs, and Enhance the Over-all maintainability and trustworthiness of one's code.
Imagine Like a Detective
Debugging is not only a specialized undertaking—it is a form of investigation. To efficiently discover and take care of bugs, builders have to tactic the procedure like a detective solving a mystery. This attitude will help stop working advanced challenges into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs and symptoms of the issue: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect just as much applicable information and facts as you can without leaping to conclusions. Use logs, check instances, and user reports to piece together a transparent photograph of what’s occurring.
Following, kind hypotheses. Request on your own: What may very well be resulting in this conduct? Have any adjustments lately been produced to the codebase? Has this difficulty happened ahead of underneath related conditions? The aim would be to slender down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation inside of a managed setting. Should you suspect a specific functionality or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code issues and Allow the results guide you closer to the reality.
Pay out close awareness to tiny details. Bugs typically conceal in the minimum predicted areas—like a missing semicolon, an off-by-1 error, or even a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes might disguise the real difficulty, just for it to resurface later.
And finally, keep notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for potential problems and support Many others realize your reasoning.
By wondering like a detective, developers can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering concealed issues in sophisticated programs.
Generate Tests
Creating exams is among the simplest tips on how to enhance your debugging expertise and overall improvement efficiency. Exams not merely enable capture bugs early but will also function a security Web that offers you self-assurance when making changes for your codebase. A effectively-examined application is easier to debug since it permits you to pinpoint just the place and when a challenge takes place.
Start with device checks, which deal with individual capabilities or modules. These compact, isolated checks can promptly expose irrespective of whether a selected bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know in which to search, substantially decreasing the time used debugging. Device assessments are In particular valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Following, integrate integration checks and conclusion-to-stop tests into your workflow. These help make sure that various portions of your application work alongside one another efficiently. They’re especially practical for catching bugs that arise in complicated units with a number of components or solutions interacting. If a little something breaks, your exams can tell you which Component of the pipeline failed and underneath what situations.
Crafting assessments also forces you to definitely Consider critically regarding your code. To test a characteristic properly, you require to know its inputs, predicted outputs, and edge instances. This standard of comprehending The natural way prospects to raised code structure and less bugs.
When debugging a difficulty, producing a failing test that reproduces the bug might be a robust first step. After the exam fails regularly, you may concentrate on repairing the bug and enjoy your test move when The difficulty is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the frustrating guessing sport into a structured and predictable course of website action—encouraging you catch far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the issue—looking at your display for hrs, hoping Alternative after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your mind, reduce frustration, and often see the issue from the new standpoint.
If you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your Mind gets less economical at trouble-fixing. A short walk, a coffee crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through more time debugging sessions. Sitting down in front of a display, mentally stuck, is not simply unproductive but in addition draining. Stepping absent means that you can return with renewed Vitality and a clearer way of thinking. You could possibly all of a sudden see a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to maneuver all around, stretch, or do a thing unrelated to code. It may experience counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise tactic. It gives your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Understand From Each individual Bug
Each and every bug you face is more than just A brief setback—It is really an opportunity to develop being a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something useful in case you make the effort to replicate and analyze what went Mistaken.
Start out by inquiring you a few important queries after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught before with superior tactics like device tests, code assessments, or logging? The responses normally reveal blind spots inside your workflow or comprehending and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. After a while, you’ll start to see patterns—recurring challenges or prevalent faults—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually In particular strong. Whether or not it’s through a Slack concept, a short create-up, or A fast expertise-sharing session, aiding others steer clear of the identical issue boosts workforce effectiveness and cultivates a stronger Finding out tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as vital parts of your progress journey. In spite of everything, a few of the most effective developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, Every bug you correct provides a fresh layer to your ability established. So next time you squash a bug, take a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — however the payoff is big. It will make you a more effective, self-confident, and able developer. The next time you're knee-deep in the mysterious bug, recall: debugging isn’t a chore — it’s a chance to be superior at what you do.